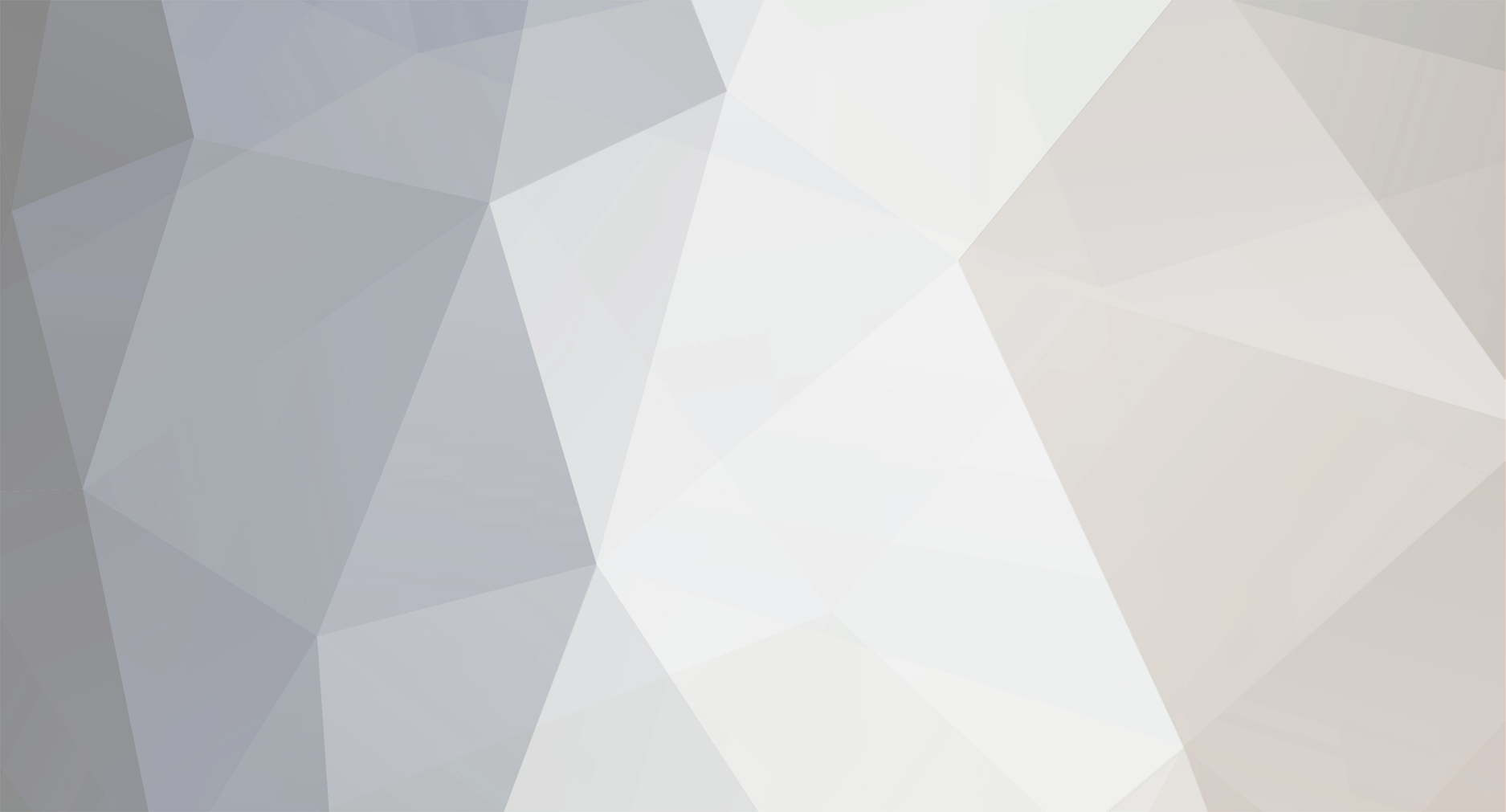
feudalnate
Members-
Posts
21 -
Joined
-
Last visited
Everything posted by feudalnate
-
It was to explain (code-wise) why when you select 'flash from CD-ROM' it errors out, not a suggestion to use that method There can be any number of reasons why the chip won't write properly. Bad flash chip, something wrong with the CPLD or the code it's running, bad connection somewhere/fatigued solder joint, bad cable, bad switch, one of the pins/wires to the LPC port is bad, or even that FlashBIOS 3.0.3 doesn't support X2 chips because I was under the impression that FlashBIOS 2.6 was for X2 and FlashBIOS 3.X was for the X3 chips but I'm unsure
-
Flashing from DVD-ROM isn't going to work unless you have a disc inserted with known BIOS image names present on it and if part of the screen wasn't cut off you could see that the flash from HDD/HTTP is failing because the erase command is failing My guess is the chip thinks flash protect is enabled - could be caused by the switch being wired backwards (seen it before), bad connection on dip switch board, bad dip switch cable, or something faulty on the chip itself Try the flash protect in the opposite direction, boot into FlashBIOS, switch the bank switch to bank1, and then write an 256K image from HDD or over HTTP. If that doesn't work, you can try booting into FlashBIOS and then unplugging the dip switch board - although, I have no idea what the default behavior of that chip is when the board is unplugged. Good luck
-
Cromwell might boot without a valid EEPROM or could be edited to do so. It's likely some of the 4th generation modchips with built-in settings/recovery menus could boot into their respective menu as well Retail/debug kernels (including modded versions) will panic shortly into initialization without valid EEPROM data, no matter the console revision. 1.6/1.6B revisions won't make it past the bootloader without valid EEPROM data
-
Week or so back I was doing some testing with various dashupdate.xbe files extracted from some early Xbox LIVE enabled games (Xbox LIVE enabled games were always shipped with a dashboard updater) and ended up finding quite a few different dashboard/online dashboard revisions that I haven't seen listed anywhere, which got me curious about how many dashboard revisions were actually pushed out (on disc, can't exactly know which were pushed over Xbox LIVE anymore) I was looking for a list of Xbox LIVE enabled games and was surprised that couldn't find one anywhere. There are plenty of lists for Xbox games but none seemed to note whether a game supported any Xbox LIVE functionality or not, so before I could look more into the dashboard updaters I had to make a list of games that actually contain them While it's not exactly the most useful list to have anymore, I still think it's something worth archiving and I'm posting this thread to see if others would be interested in helping Personal spreadsheet (my personal working doc, read-only to public) Public spreadsheet (public doc, anyone is free to add to it or edit it) References I've used building this list: Xbox.com (archived, for game XBL feature list) Wikipedia (for initial release dates) mobygames.com (for release dates and back covers with feature list) xboxaddict.com (for release dates and back covers with feature list) gamespy.com (for release dates, sometimes features list) gamescanner.org (archived, back covers with feature list) xbmcxbox blog (grabbed list of games that all contain an uninteresting dashboard revision) ebay.com (back covers with feature list, more useful than you might think) Whether people help or not is up to them but I'll continue to flush out the list. If you want to post the name of a game in this thread that you know has some kind of Xbox LIVE functionality and isn't already on this list then that's fine too
-
- [research]
- xbox
-
(and 3 more)
Tagged with:
-
When this guy said "it's my softmod that is preventing the 576i capability" I immediately thought of this guy that wouldn't accept advice about XboxHDM and responded about as well as this guy does. I had to promptly check out of this "conversation". Good luck Dave, if you're brave enough to stick around for this one
-
Yes, your logic is truly flawless and there is absolutely no technical counter argument that anyone in existence could make. Good luck!
-
The Xbox doesn't support 50hz output over component. 480p@60hz, 720p@60hz, and 1080i@30hz are the only supported video modes over component. The Xbox will boot with the manufacturing set video mode and settings before switching to what's supported by the cable it detects and attempts to apply user set video settings. Standard AV, SCART/Advanced SCART, and RF were the only officially supplied and supported video cables for PAL region consoles - but you would know this if you learned to read, since this is clearly stated in the Xbox manual.
-
TjMax for the old Pentium III CPU's is around 85c officially. From what I've witnessed on the original Xbox, at around 82-83c the SMC will power off the console and blink the overheated LED error code. The other temperature reading is for ambient case temp. ("air" temp.), which seemingly has no purpose If your CPU temp. is sitting 10-15c below max while gaming then there's no real issue. Almost everything on the Xbox runs as a 3D application just like games, there's no true "idle" state to compare against If you're concerned with the temperature then you can turn up your fan speed and trade noise for heat reduction but I'm going to agree with Dave and say ~70c coming out of a game is okay
-
Research into the Xbox EEPROM data
feudalnate replied to feudalnate's topic in General Xbox Discussion
For the older revisions of 2BL there are similar tables of values that are stored and read directly from the BIOS image, sent to the NB/GPU to do calibration and is immediately followed by the memory test. I don't think you're far off on your guess, it's just the strange thing is that the table was moved into the EEPROM instead when older revision BIOS images have a specific table for Samsung memory and another for Micron memory then just does a check beforehand to see what type of memory the system has and uses the appropriate one but in the case of 1.6 and 1.6B models they shoved the table into the EEPROM instead It's like they didn't know if they could get different memory chips in advance when releasing 1.6 revisions? It would have been trivial for them to just update the table in the BIOS and recompile it or just have multiple tables stored for different types of memory like the earlier revisions. Not sure, maybe they manufactured all the 1.6 boards at the same time with the standard 1.6 BIOS pre-flashed to the ROM in Xyclops chip and then just used whatever memory they had on hand then updated the hardware section of the EEPROM accordingly? I stated in my write up that perhaps it had something to do with the model of GPU they used and that might be true too, it's difficult to say. The 1.6 revision boards have always seemed a bit hacky and probably why it has various issues with even retail software I can confidently say that those values in the hardware section of the EEPROM are for memory but I have no idea how those values work when they're passed to the GPU BIOS -
Research into the Xbox EEPROM data
feudalnate replied to feudalnate's topic in General Xbox Discussion
For those that are interested I have written some documentation on the EEPROM. There's still a bit of research to do but for the most part it's complete and as far I can tell, is the most robust documentation on the subject. Let me know if you spot any mistakes/misinformation or something I missed https://github.com/feudalnate/Original-Xbox-Data-Structures/blob/master/EEPROM/README.md I would have edited this into my original post but this usergroup doesn't have edit permissions -
For the last year or two I've been working on reversing some of the data structures that have been left unresearched or incomplete by the old scene, not to such a hardcore degree as I would honestly like but off and on when I have the time. This spans from what most people have seen me put out like the Xbox LIVE account stuff but I also have an interest in FATX16/32 structuring, the various configuration/datastore sectors at the beginning of the Xbox hard drive, the 1BL/FBL/2BL/BIOS image structures and data packing, XBE header/certificate data, security, and structuring, and the EEPROM data and structuring - the fundamental data types that make up the Xbox Today I completed the EEPROM data structure - well I say "completed" but there are still some things like flags, bitpacking, and a single unlabeled 16bit value to truly finish up all the info on the data handling side of things - however, structurally I have mapped out all the EEPROM data including the notorious hardware section as well as reversing the XConfigChecksum into something much more legible, portable, and simply more correct than what's been sitting on the XboxLinux/XboxDev wiki's for the last decade and a half I didn't really feel posting something like this on Reddit would be the way to go, the constant Twitter-feed like nature of threads just buries information and I have always preferred forums for their organization - and besides, forums are where I started in modding and forums are what I ran/helped run for many years of my modding life Anyway to my point, if you're interested in the structure and/or (partial for now) handling of EEPROM data then I've posted all the info on my GitHub project. This information can be used to parse, edit, or build an EEPROM image from scratch for any revision of the Xbox (including 1.6 and 1.6b models). I'll likely make a tool for people to create/edit EEPROM images at some point but I've got a couple Xbox projects I've been working on for a while and would like to finish at least one of those before starting another and I'll stuff all this structuring information into a table on the project page soon or maybe edit the XboxDev wiki so it's easier for non-programming people to view and understand at a glance Thanks for reading (also, posting this in the general forum wasn't my first choice but this doesn't really fit into the topic of homebrew - it's more so R&D) This paragraph has nothing to do with the subject of my post but I just wanted to say something personal here. I post this kind of information and put out these various tools and answer these questions that most people don't have an answer to for the sole purpose of bettering or further educating and simply just passing on information I know to the few left in original Xbox scene and even though I might come off as such it's never really been an ego or "clout" thing for me with the original Xbox, I've owned an original Xbox since 2001 and it's purely a passion thing. All I'm really after with this hobby of research and modding of the Xbox is to leave the scene with correct information or at least as correct as I'm able to comprehend and express to others, which I'm sure the old members of the early scene tried to do as well. The reason the original Xbox still lives on is because of sites like this and the handful of people that still hold onto their interest of the original Xbox or their passion of tinkering. Some of you may know my username and others may not, I've seen people mention me as this sort of Xbox "guru" or "expert" and I don't know if I come across as such but I can say, there are many things I don't know, many things I feel I'll never know, and a lot of times I feel like I barely know anything but I know objectively this isn't entirely true because there's a lot I know about the Xbox (and Xbox 360 for that matter) and yet it doesn't stop me from feeling dumb much of the time. Everything I know about computers, the Xbox/Xbox 360, data, structuring, programming, etc. is all self-taught and if you want to learn the type of stuff I know then you absolutely can teach yourself these things and it doesn't necessarily need to be on the software side of things, if you want to know hardware then you can teach yourself that as well - push yourself to learn, you are your best teacher. If you're part of the Xbox scene, whether you're a complete noob or have been around forever, whether we've had good/bad interactions in the past or maybe in the future, know that I appreciate you regardless of your knowledge or "status" and know that I hold no negative feelings towards anyone left in this scene and I probably never will. Not entirely sure why I just wrote this rant but hopefully no one minds, I'm just glad I'm not alone in this hobby of playing around with a 20 year old outdated machine
-
When you tried XBOXSCENE and TEAMASSEMBLY did you pad to 32 bytes? Unlocking with the master password will only temporarily disable security on the drive, if the user password is set (and it is on the Xbox) then only the user password can disable the security state and have it persist through a power cycle. If you are able to unlock the drive with the master password you will need to copy everything off the drive and then send a secure erase command - this will wipe the drive and disable security Try these master passwords //XBOXSCENE 58424F585343454E450000000000000000000000000000000000000000000000 //XBOX-SCENE 58424F582D5343454E4500000000000000000000000000000000000000000000 //TEAMASSEMBLY 5445414D415353454D424C590000000000000000000000000000000000000000 Most UDE2/UXE softmod installers will set one of these as the master password. Here is documentation on ATA/APAPI security states and commands
-
research 'Market Research' into Users Preferred BIOSes to use.
feudalnate replied to empyreal96's topic in Bios
EvoX M8/M8+ is the most common BIOS used for modchips, TSOP, and softmods (PBL) iND 5003/5004 is somewhat common, usually used by people that don't want to use M8/M8+ or people that need it for it's 128MB memory support or ability to have an external config file X2 is seldomly used aside from people that want to use it with an X2 chip or people that need it for it's 128MB memory support or ability to have an external config file M7 and below is usually used by people that have an old chip/TSOP that they don't know how to update or care to update X3 is used by people that have an X3 chip because of it's embedded configuration/recovery program Debug BIOS' are generally used only by programmers, curious people, or someone using an XDK When you ask for "BIOS revision" what are you referring to? If you mean a retail image then there's only 2 revisions of the bootloader(s) (1.0, 1.1-1.6) - there are variables and offsets that are changed between 1.1-1.6 models but they are more or less exactly the same and not enough is changed to be classified as their own BIOS revision - else if you're talking kernels then there are many Size of a retail BIOS image is always 256KB and the one existing debug BIOS is 512KB. 1.0 consoles have the BIOS duplicated 4 times to fill a 1MB area I suppose the 1.1 MCPX is more common since it was used in more revisions of the motherboard (1.1 and onward use the 1.1 MCPX), the 1.0 revision motherboard is the only revision that uses the 1.0 MCPX southbridge chip. This is more of a production quantity and sales question, not really a question anyone in the Xbox scene can answer Some of the questions you ask have answers with a lot of variables to them, I don't believe anyone left in this scene could answer them all concretely. If you're researching the Xbox BIOS then XboxDevWiki is a good place to start, it offers information and references but there are many unknowns still to be researched- 3 replies
-
- 1
-
-
- bios
- development
- (and 7 more)
-
I'm surprised no one has mentioned recovery via modchip. Replace your current modchip with one that has an intermediate interface between startup and when a BIOS loaded, one that supports EEPROM access - a modchip such as the OpenXenium, Xenium, SmartXX, Xecuter 3, etc. can recover the EEPROM. You will need to write a 1.6 EEPROM image, if your hard drive isn't locked then the EEPROM image doesn't necessarily need to be your own but it needs to come from a 1.6 board (untested, would recommend writing the original EEPROM for the board if you have it) You can grab an OpenXenium off eBay for $25 For future reference: 1.0 EEPROM images only work on a 1.0 board 1.1-1.4(1.5?) EEPROM images only work on 1.1-1.4(1.5?) boards 1.6(b) EEPROM images only work on 1.6(b) boards 1.0-1.4(1.5?) EEPROM images can be edited to work between these models but 1.6(b) EEPROM images contain a required hardware configuration data section and must be written to 1.6(b) boards only (perhaps other model EEPROM images could be edited to add this section but it's unclear if this hardware section is board specific or not) Your mistake was writing an entire EEPROM image from another console instead of simply updating the XboxHDKey for locking
-
Why did flashing a kernel file with XBlastOS break the XBOX?
feudalnate replied to calebTree's topic in Bios
If you wrote a BIOS version of 1.0.4627.X or lower to a 1.1-1.4 motherboard or a BIOS version of 1.0.4817.X or above to a 1.0 motherboard then the result would be a "coma console" because 1.0 and 1.1+ motherboards have a differing MCPX ROM and use a different encryption algorithm for decrypting 2BL into memory (1.1-1.4 boards also have an extra step in the bootchain) All official retail Microsoft BIOS' are 256KB so if you wrote a 1MB BIOS image and it wrote at least 256KB of the image to flash then that's technically okay but if you wrote a 1.0 image to a 1.1-1.4 revision or vise-versa then 2BL can't be decrypted properly and that's why your console is 'bricked'. You can recover the console by installing a modchip and I believe you can recover the TSOP as well but I'm not the one to answer how to go about that Quick rundown of critical failure states on the Xbox: Coma console: An error in 1BL or fatal hardware failure (CPU, NB (imbedded in GPU), SB (MCPX), RAM, SMC, or TSOP, and anything in between connecting those chips.. so basically anything) - no code to be able to signify an error to a user LED blinking: An error in 2BL (or FBL/2BL on 1.1+ revisions) or fatal hardware failure (RAM, GPU, or EEPROM) or something wrong with the kernel image - can blink LED's with code to write to SMC Error screen: An error in kernel or something wrong with higher level buses, devices, software, or basically any chip on the board not behaving correctly when the console is in a fully initialized state You're correct in saying BIOS' and kernels are usually separate things, the Xbox doesn't actually have a "BIOS" but that's what the modding scene labeled it as a long time ago - it's more so firmware. The Xbox "BIOS" is 2-3 separate blobs of code mushed together into a single chunk of data stuffed onto a flash chip (or ROM on 1.6) BIOS = 2BL + kernel or BIOS = FBL + 2BL + kernel (in the case of 1.1+ revisions) -
If you're booting from a bank area that has an official X3 BIOS written to it then you can hold the white button on a controller during the boot animation and it will bring you to the X3 Config LIVE menu Here is a scrape of the user guide site. Most of the pages should be cached on archive.org as well
-
Post your save and the default.xbe for the game. Likely a signing issue, either different title key from a different region XBE or saves are locked to the per-console XboxHDKey
-
Can you expand on your reasoning a bit? Are you wanting to get device packets during arbitrary execution? Are you avoiding kernel calls? The Xbox doesn't store controller states in memory, you must request and poll peripheral states every time you want to check (SMBus->MCPX (southbridge)->USB hub (controller ports 0-4)->controller (also a USB hub)->port 0). If you want to utilize kernel driver calls and you're not compiling against the XTL then you're going to need to lookup the function addresses and the lookup must be dynamic every time because of the differing kernel versions on the Xbox. If you want to avoid the kernel then you can check how the devs that wrote the cromwell BIOS managed talking to the southbridge/USB host controller/USB devices (fairly certain they wrote multiple drivers) This is bare minimum to fetch controller states with the kernel #include <xtl.h> void WaitAnyControllerStateChange(XINPUT_STATE* ControllerState) { int Changed = 0; //Setup controller input XDEVICE_PREALLOC_TYPE DeviceTypes[] = { {XDEVICE_TYPE_GAMEPAD, 4} }; //4 = polling on all ports XInitDevices(sizeof(DeviceTypes) / sizeof(XDEVICE_PREALLOC_TYPE), DeviceTypes); //init device stack Sleep(500); //have to sleep, it takes almost half a second to initialize the XInput library.. //open handles to each port HANDLE Controllers[4]; Controllers[0] = XInputOpen(XDEVICE_TYPE_GAMEPAD, XDEVICE_PORT0, XDEVICE_NO_SLOT, 0); Controllers[1] = XInputOpen(XDEVICE_TYPE_GAMEPAD, XDEVICE_PORT1, XDEVICE_NO_SLOT, 0); Controllers[2] = XInputOpen(XDEVICE_TYPE_GAMEPAD, XDEVICE_PORT2, XDEVICE_NO_SLOT, 0); Controllers[3] = XInputOpen(XDEVICE_TYPE_GAMEPAD, XDEVICE_PORT3, XDEVICE_NO_SLOT, 0); DWORD Insertions = 0; DWORD Removals = 0; while(1) { if (XGetDeviceChanges(XDEVICE_TYPE_GAMEPAD, &Insertions, &Removals)) //check connection state changes { //something changed, refresh controller handles for(int i = 0; i < 4; i++) { if (Controllers[i] != 0) XInputClose(Controllers[i]); //close any current handles (system doesnt dealloc them itself) } //open new handles Controllers[0] = XInputOpen(XDEVICE_TYPE_GAMEPAD, XDEVICE_PORT0, XDEVICE_NO_SLOT, 0); Controllers[1] = XInputOpen(XDEVICE_TYPE_GAMEPAD, XDEVICE_PORT1, XDEVICE_NO_SLOT, 0); Controllers[2] = XInputOpen(XDEVICE_TYPE_GAMEPAD, XDEVICE_PORT2, XDEVICE_NO_SLOT, 0); Controllers[3] = XInputOpen(XDEVICE_TYPE_GAMEPAD, XDEVICE_PORT3, XDEVICE_NO_SLOT, 0); } //poll controller states on all ports for(int i = 0; i < 4; i++) { if (Controllers[i] != 0) //check bad handle { if (XInputGetState(Controllers[i], ControllerState) == ERROR_SUCCESS) //check input state changes { Changed = 1; break; //something changed, return state } } } if (Changed) break; } } void main() { XINPUT_STATE ControllerState = { 0 }; WaitAnyControllerStateChange(&ControllerState); /* process state data... */ } No one is going to be able to give you some quick code to poll controller state because it's not a simple thing to do
- 4 replies
-
- controller
- status
-
(and 1 more)
Tagged with:
-
Question About the Dangers of Nulling the Hdd Key.
feudalnate replied to bulkchart32's topic in General Xbox Discussion
Anywhere the Xbox/Xbox 360 modding scene is you will likely find me even if I haven't posted anything, I'm usually around. The unknowns and obscure things are what still keeps my interest in the Xbox modding scenes I don't really disagree with any of this, DLC/updates can be signed to the new key fairly easily (shameless plug: https://github.com/feudalnate/XBX-Content-Tool) but gamesaves are more specific and some of those saves that use non-roamable signing can even incorporate scrambling/encryption which makes those saves that much more difficult to recover (although this type data security doesn't seem too common) I don't have anything against those that want to change their console keys but I also don't get why holding onto your original EEPROM data presents any sort of difficulty. You mention your scenario dealing with many consoles and I can see it how having all those consoles sharing the same key can be beneficial to someone dealing with the same situation but most people aren't going to share this type of situation. The average Xbox fan/enthusiast is going to have one or two consoles and holding onto their consoles EEPROM data isn't much of a problem My consensus on this is that people can do whatever they please with their consoles and I don't disagree with the convenience and ease of recovery that setting the XboxHDKey to something simple to remember can provide I have a couple of those discs laying around myself from the early days of modding. The problem with running things like this in Windows is that you're running in user-mode and Windows doesn't like when random programs send direct ATA commands to devices - programmers kind of need to fight with this to get Windows to play nice and that's why running the old style of XBHDM on boot to bypass Windows generally works better From what I've seen it's mostly games that were released after modding was known to be rampant (2003-2004?). Can't provide a specific list of games, really all I can say on this but there's a good amount Hard drive The most commonly used functions in homebrew programs that deal with ATA security commands come from an open source library that Yoshihiro/Team-Assembly put out in ~2003. The functions for sending ATA commands for disabling and setting the password are very hacky and they do work.. technically. The issue with these widely used functions is that they do not follow the ATA specification at all and that is what is dangerous There is no check for the device ready flag to know if the command sent will be accepted. The "cleaning" of model/serial characters manually pulled from an identify packet command instead of pulling and using already prepared data sitting in the kernel, the exact data the kernel requires and will expect when generating the hard drive password. Commands being directly sent to the I/O port instead of through the DeviceIoControl function in the kernel that can provide additional context setup, mutexing, and checking. No checking for error flags in the error register after a command is sent even though direct port access is being used. The sending of ATA commands, looping for X amount of tries until successful but always returning true even if the command was in fact, not successful EEPROM Programming any type of flash while in-circuit carries an inherit risk. It's much the same risk as writing a TSOP or a cheapmod modchip with no recovery software in place. I'm sure there's a more technical term for this type of IC writing but I've always referred to it as a kamikaze write - where if anything were to be written incorrectly for any reason then that IC will be left in a bricked state I'll say this, there is a reason Microsoft immediately pulls all EEPROM data into memory upon powering on the console, leaves it there in memory for the entire duration the console is powered on, and always references that copy in memory instead of directly accessing the IC. There is a reason most kernel functions specifically avoid writing to the EEPROM and only do so when there is a setting that must persist through a power cycle. There is a reason why a bunch of non-vital settings in the EEPROM go unused in retail kernels and a reason why those settings were moved into the "config" area on the hard drive instead A lot of the homebrew programs that deal with these two security areas on the Xbox do usually work and I won't say they don't but there is always a chance of failure in the programming of these homebrew programs and to say that there isn't would be ignorant of reality. I'm sure many Xbox's have "died" to an error during EEPROM writes, not only from homebrew software but from the Xbox/Xbox LIVE dashboards as well. Just because no one specifically reports an EEPROM write error doesn't imply it's not a real thing and most people aren't going to know this was what caused their issue to begin with so how would they report it. However, an incorrectly set security state for the hard drive will always come from homebrew of some kind Even when done correctly, dealing with these security areas is risky and the risk is there because both the EEPROM and the hard drive are critical system components As I said in my initial post, a zeroed XboxHDKey is not considered valid by the kernel. The kernel doesn't throw an error for this but if you were to somehow attach a debugger to a retail console while it boots, it would assert an error on kernel initialization. A zeroed XboxHDKey is only valid on debug kernels Realistically if this new homebrew LIVE service comes to light then the real issue looking forward is how is the LIVE dashboard going to function when softmods based on the UXE exploit use an exploitable version of the LIVE dashboard? How are softmods going to boot when they require the LIVE dashboards files but so does this homebrew service? Will need to see how things turn out with this homebrew LIVE service first to see if a solution is needed - the XboxHDKey is only one problem of potentially many Some references ATA/ATAPI-4 Specification ATA Security Clarification XKUtils Library by Yoshihiro/Team-Assembly Most of what I've stated in this thread stems from research I've done with the kernel, EEPROM, SMC, and hard drive (along with looking into open source homebrew, reversing homebrew, and softmod stuff) -
Question About the Dangers of Nulling the Hdd Key.
feudalnate replied to bulkchart32's topic in General Xbox Discussion
There are quite a few consequences and risks to changing the XboxHDKey Any currently installed downloadable content and updates become invalid Any gamesaves signed as "non-roamable" become invalid Any Xbox LIVE accounts stored on the hard drive become invalid If Xbox LIVE accounts were stored on the hard drive then the machine account becomes invalid as well A zeroed (nulled) XboxHDKey on a retail console is not considered valid (however is completely valid on XDK) There are several risks in the process of changing the XboxHDKey as well, especially on softmodded systems and cheapmod modchips with no intermediate recovery menu Failing to write the EEPROM data back properly The SMC failing in the middle of a write transaction could corrupt the EEPROM, resulting in a "bricked" EEPROM Homebrew failing to encrypt the 'EncryptedSection' of the EEPROM data properly (where the XboxHDKey is stored), resulting in a "bricked" EEPROM Homebrew failing to check for a successful EEPROM write before changing the hard drive password, which could result in the hard drive being locked with an incorrect password Homebrew failing to check if ATA commands to the hard drive for disabling password/setting user password were successful after already writing EEPROM changes, which could result in the hard drive being in a permanently unlocked state or leaving the hard drive locked with the old password Homebrew failing to generate the hard drive password correctly and setting an improper password, leaving the system in an unbootable/inoperable state Homebrew failing to roll back changes in the event of an error if either the process of EEPROM I/O or ATA I/O fails, leaving the system in an unbootable/inoperable state Homebrew (or even retail software) crashing during either the process of EEPROM I/O or ATA I/O, leaving the system in an unbootable/inoperable state Zeroing the XboxHDKey makes recovery easier in the event of a failed hard drive sure but is simply keeping a backup of the original EEPROM data and leaving the XboxHDKey as-is really that much more difficult? Never really understood people always suggesting zeroing this EEPROM setting but then again, those same people never seem to explain the reasons why doing so can be detrimental and carry heavy risk I still have my EEPROM backups from 2002 ¯\_(ツ)_/¯
Board Life Status
Board startup date: April 23, 2017 12:45:48